This set of MCQ(multiple choice questions) focuses on the Problem Solving Through Programming In C Week 6 Solutions.
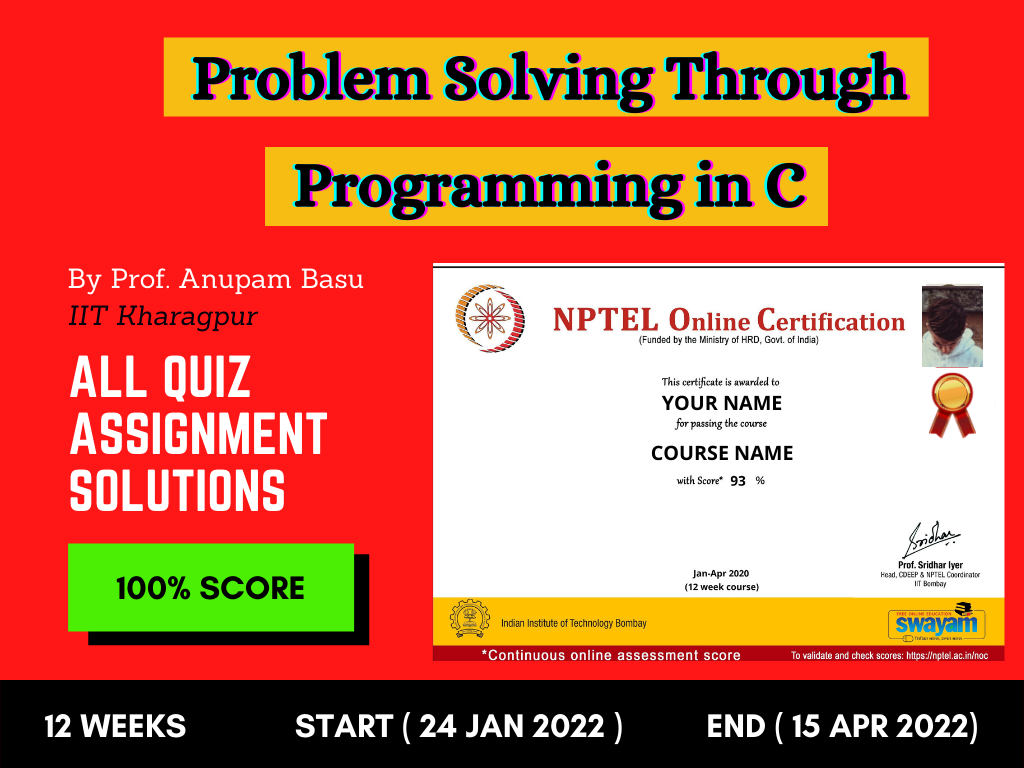
Course layout (Answers Link)
Answers COMING SOON! Kindly Wait!
Week 0 : Assignment
Week 1 :Â Introduction to Problem Solving through programs
Week 2 :Â Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching
Week 3 :Â Conditional Branching and Iterative Loops
Programming Assignment
Week 4 :Â Arranging things : Arrays
Programming Assignment
Week 5 :Â 2-D arrays, Character Arrays and StringsÂ
Programming Assignment
Week 6 :Â Basic Algorithms including Numerical Algorithms
Week 7 :Â Functions and Parameter Passing by Value
Week 8 :Â Passing Arrays to Functions, Call by Reference
Programming Assignment
Week 9 :Â Recursion
Week 10 :Â Structures and Pointers
Week 11 :Â Self-Referential Structures and Introduction to Lists
Week 12 :Â Advanced Topics
NOTE: You can check your answer immediately by clicking show answer button. Problem Solving Through Programming In C Week 6 answers” contains 10 questions.
Now, start attempting the quiz.
Problem Solving Through Programming In C Week 6 answers
Q1. What is an array in C?
a) A collection of similar data elements with the same data type.
b) A built-in function that performs mathematical calculations.
c) A keyword used for declaring variables.
d) A data type used to store characters only.
Answer: a) A collection of similar data elements with the same data type.
Q2. What is the index of the first element in an array?
a) 0
b) 1
c) -1
d) The index can vary depending on the array size.
Answer: a) 0
Q3. Which loop is commonly used to iterate through all elements of an array in C?
a) for loop
b) while loop
c) do-while loop
d) switch loop
Answer: a) for loop
Q4. An integer array of 15 elements is declared in a C program. The memory location of the first byte of the array is 2000. What will be the location of the 13th element of the array? Assume int takes 2 bytes of memory.
a) 2013
b) 2024
c) 2026
d) 2030
Answer: b) 2024
Q5. How can you find the sum of all elements in a 1D array “arr” with 5 elements using loop in C?
a) sum = arr[0] + arr[1] + arr[2] + arr[3] + arr[4]
b) sum = arr[5]
c) for(int i=0; i<=5; i++) { sum += arr[i]; }
d) for(int i=0; i<5; i++) { sum += arr[i]; }
Answer: d)
Q6. What is the output of the following code?
a) 1 3 5
b) 1 2 3 4 5
c) 1 2 3
d) 1 4
Answer: a) 1 3 5
Q7. What will be the output?
a) i=5, j=5, k=2
b) i=6, j=5, k=3
c) i=6, j=4, k=2
d) i=5, j=4, k=2
Answer: a) i=5, j=5, k=2
Q8. What will be the output after execution of the program?
a) 1
b) 2
c) 3
d) 4
Answer: d) 4
Q9. What will be the output?
Answer: 8
Q10. Find the output of the following C program.
a) 5, 4
b) 5, 5
c) 4, 4
d) 3, 4
Answer: c) 4, 4
Problem Solving Through Programming In C Week 6 answers
Q1. What is the right way to initialise an array in C?
a) int arr{} = {1,2,5,6,9}
b) int arr[5] = {1,2,5,6,9}
c) int arr{5} = {1,2,5,6,9}
d) int arr() = {1,2,5,6,9}
Answer: b) int arr[5] = {1,2,5,6,9}
Q2. An integer array of dimension 10 is declared in a C program. The memory location of the first byte of the array is 1000. What will be the location of the 9th element of the array?
a) 1028
b) 1032
c) 1024
d) 1036
Answer: b) 1032
Q3. What will be the output after execution of the program?
a) 1
b) 2
c) 3
d) 4
Answer: a) 1
Q4. Which of the statements is/are correct?
a) An array may contain more than one element
b) All elements of array have to be of same data type
c) The size of array has to be declared upfront
d) All of the above
Answer: d) All of the above
Q5. What actually gets passed when you pass an array as an argument to a function
a) Value of elements in array
b) First element of the array
c) Base address of the array
d) Address of the last element of array
Answer: c) Base address of the array
Q6. Find the output of the following C program
a) 5, 4
b) 5, 5
c) 4, 4
d) 3, 4
Answer: c) 4, 4
Q7. What will be the output?
a) 5
b) 6
c) 9
d) 10
Answer: c) 9
Q8. An array of the void data type
a) can store any data-type
b) only stores element of similar data type to first element
c) acquires the data type with the highest precision in it
d) It is not possible have an array of void data type
Answer: d) It is not possible have an array of void data type
Q9. What will be the output?
Answer: 20
Q10. How many ‘a’ will be printed when the following code is executed?
Answer: 6
Previous Course – Week 6 assignment answers
Q1. Which of the following is correct statement to access 5th element in a array arr[] of size 50?
a) arr[5]
b) arr[4]
c) arr{5}
d) arr{4}
Answer: b) arr[4]
Q2. Which statement is correct?
a) An index or subscript in array is a positive integer
b) An index or subscript in array is a positive or negative integer
c) An index or subscript in array is a real number
d) none of the above statement are correct
Answer: a) An index or subscript in array is a positive integer
Q3. What is the output of C Program?
#include<stdio.h>
int main()
{
int a[3] = {10, 12, 14};
a[1] = 20;
int i = 0;
while(i<3)
{
printf("%d ", a[i]);
i++;
}
return 0;
}
a) 20 12 14
b) 10 20 14
c) 10 12 20
d) Compiler error
Answer: b) 10 20 14
Q4. An integer array (An integer takes two bytes of memory) of size 15 is declared in a C program. The memory location of the first byte of the array is 2000. What will be the location of the 13th element of the array?
a) 2013
b) 2024
c) 2026
d) 2030
Answer: b) 2024
Q5. To compare two arrays, we can do it by using
a) comparison operator ‘==’ directly on arrays
b) ‘ switch case’
c) ‘for’ loop
d) ‘ternary operator’ on arrays
Answer: c) ‘for’ loop
Q6. How many ‘a’ will be printed when the following code is executed?
#include<stdio.h>
int main()
{
int i = 0;
char c = 'a';
while(i<5)
{
i++;
switch(c)
{
case 'a': printf("%c ", c);
break;
}
}
printf("a\n");
return 0;
}
Answer: 6
Q7. What is the output of the following C program?
#include<stdio.h>
int main()
{
int arr[2] = {1, 2, 3, 4, 5};
printf("%d", arr[3]);
return 0;
}
a) 3
b) 4
c) No output
d) Compilation error
Answer: d) Compilation error
Q8. What will be printed after execution of the following code?
#include<stdio.h>
int main()
{
int arr[2] = {1, 2, 3, 4, 5};
printf("%d", arr[5]);
return 0;
}
a) Garbage value
b) 0
c) 5
d) 6
Answer: b) 0
Q9. What is the output of the following C code?
#include<stdio.h>
int main()
{
int i;
int arr[3] = {1};
for(i=0; i<3; i++)
printf("%d", arr[i]);
return 0;
}
a) 1 followed by two garbage values
b) 1 1 1
c) 1 0 0
d) 0 0 0
Answer: c) 1 0 0
Q10. What will be the output when the following code is executed.
#include<stdio.h>
int main()
{
int arr[6] = {1, 2, 3, 4, 5, 6};
switch(sizeof(a))
{
case 1:
case 2:
case 3:
case 4:
case 5:
printf("IIT KGP);
break;
}
printf("IIT MADRAS");
return 0;
}
a) IIT KGP
b) IIT MADRAS
c) Compilation error
d) Nothing is printed
Answer: b) IIT MADRAS
<< Prev – An Introduction to Programming Through C Week 5 Solutions
>> Next- An Introduction to Programming Through C Week 7 Solutions
DISCLAIMER: Use these answers only for the reference purpose. Quizermania doesn't claim these answers to be 100% correct. So, make sure you submit your assignments on the basis of your knowledge.
For discussion about any question, join the below comment section. And get the solution of your query. Also, try to share your thoughts about the topics covered in this particular quiz.